Prometheus (Stats)
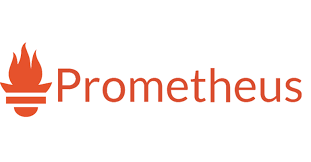
Introduction
Prometheus is a monitoring system that collects metrics, by scraping exposed endpoints at regular intervals, evaluating rule expressions. It can also trigger alerts if certain conditions are met.
OpenCensus Java allows exporting stats to Prometheus by means of the Prometheus package io.opencensus.exporter.stats.prometheus
For assistance setting up Prometheus, Click here for a guided codelab.
Creating the exporter
To create the exporter, we’ll need to:
- Import and use the Prometheus exporter package
- Define a namespace that will uniquely identify our metrics when viewed on Prometheus
- Expose a port on which we shall run a
/metrics
endpoint - With the defined port, we’ll need a Promethus configuration file so that Prometheus can scrape from this endpoint
Add this to your pom.xml
file:
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<opencensus.version>0.15.0</opencensus.version> <!-- The OpenCensus version to use -->
</properties>
<dependencies>
<dependency>
<groupId>io.opencensus</groupId>
<artifactId>opencensus-api</artifactId>
<version>${opencensus.version}</version>
</dependency>
<dependency>
<groupId>io.opencensus</groupId>
<artifactId>opencensus-impl</artifactId>
<version>${opencensus.version}</version>
</dependency>
<dependency>
<groupId>io.opencensus</groupId>
<artifactId>opencensus-exporter-stats-prometheus</artifactId>
<version>${opencensus.version}</version>
</dependency>
<dependency>
<groupId>io.prometheus</groupId>
<artifactId>simpleclient_httpserver</artifactId>
<version>0.3.0</version>
</dependency>
</dependencies>
We also need to expose the Prometheus endpoint say on address “localhost:8888”.
package io.opencensus.tutorial.prometheus;
import io.opencensus.exporter.stats.prometheus.PrometheusStatsCollector;
import io.prometheus.client.exporter.HTTPServer;
public class PrometheusTutorial {
public static void main(String ...args) {
// Register the Prometheus exporter
PrometheusStatsCollector.createAndRegister();
// Run the server as a daemon on address "localhost:8888"
HTTPServer server = new HTTPServer("localhost", 8888, true);
}
}
and then for our corresponding prometheus.yaml
file:
global:
scrape_interval: 10s
external_labels:
monitor: 'demo'
scrape_configs:
- job_name: 'demo'
scrape_interval: 10s
static_configs:
- targets: ['localhost:8888']
Running Prometheus
And then run Prometheus with your configuration
prometheus --config.file=prometheus.yaml
Viewing your metrics
Please visit http://localhost:9090
Project link
You can find out more about the Prometheus project at https://prometheus.io/